推荐使用 SignalR 来平替 WebSocket,参考教程。
【服务端(.NET)】
一、创建 WebSocketHandler 类,用于处理客户端发送过来的消息,并分发到其它客户端
public class WebSocketHandler
{
private static List<WebSocket> connectedClients = [];
public static async Task Handle(WebSocket webSocket)
{
connectedClients.Add(webSocket);
byte[] buffer = new byte[1024];
WebSocketReceiveResult result = await webSocket.ReceiveAsync(new ArraySegment<byte>(buffer), CancellationToken.None);
while (!result.CloseStatus.HasValue)
{
string message = Encoding.UTF8.GetString(buffer, 0, result.Count);
Console.WriteLine($"Received message: {message}");
// 处理接收到的消息,例如广播给其他客户端
await BroadcastMessage(message, webSocket);
result = await webSocket.ReceiveAsync(new ArraySegment<byte>(buffer), CancellationToken.None);
}
connectedClients.Remove(webSocket);
await webSocket.CloseAsync(result.CloseStatus.Value, result.CloseStatusDescription, CancellationToken.None);
}
private static async Task BroadcastMessage(string message, WebSocket sender)
{
foreach (var client in connectedClients)
{
if (client != sender && client.State == WebSocketState.Open)
{
await client.SendAsync(Encoding.UTF8.GetBytes(message), WebSocketMessageType.Text, true, CancellationToken.None);
}
}
}
}
二、在 Program.cs 或 Startup.cs 中插入:
// 添加 WebSocket 路由
app.UseWebSockets();
app.Use(async (context, next) =>
{
if (context.Request.Path == "/chat")
{
if (context.WebSockets.IsWebSocketRequest)
{
WebSocket webSocket = await context.WebSockets.AcceptWebSocketAsync();
await WebSocketHandler.Handle(webSocket);
}
else
{
context.Response.StatusCode = 400;
}
}
else
{
await next();
}
});
这里的路由路径可以更改,此处将会创建一个 WebSocket 连接,并将其传递给 WebSocketHandler.Handle 方法进行处理。
也可以用控制器来接收 WebSocket 消息。
【客户端(JS)】
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0" />
<title>WebSocket 示例(JS + ASP.NET)</title>
<style>
#list { width: 100%; max-width: 500px; }
.tip { color: red; }
</style>
</head>
<body>
<h2>WebSocket 示例(JS + ASP.NET)</h2>
<textarea id="list" readonly rows="20"></textarea>
<div>
<input type="text" id="msg" />
<button onclick="fn_send()">发送</button>
<button onclick="fn_disconnect()">断开</button>
</div>
<p id="tip_required">请输入要发送的内容</p>
<p id="tip_closed">WebSocket 连接未建立</p>
<script>
var wsUrl = "wss://" + window.location.hostname + ":" + window.location.port + "/chat";
var webSocket;
var domList = document.getElementById('list');
// 初始化 WebSocket 并连接
function fn_ConnectWebSocket() {
webSocket = new WebSocket(wsUrl);
// 向服务端发送连接请求
webSocket.onopen = function (event) {
var content = domList.value;
content += "[ WebSocket 连接已建立 ]" + '\r\n';
domList.innerHTML = content;
document.getElementById('tip_closed').style.display = 'none';
webSocket.send(JSON.stringify({
msg: 'Hello'
}));
};
// 接收服务端发送的消息
webSocket.onmessage = function (event) {
if (event.data) {
var content = domList.value;
content += event.data + '\r\n';
domList.innerHTML = content;
domList.scrollTop = domList.scrollHeight;
}
};
// 各种情况导致的连接关闭或失败
webSocket.onclose = function (event) {
var content = domList.value;
content += "[ WebSocket 连接已关闭,3 秒后自动重连 ]" + '\r\n';
domList.innerHTML = content;
document.getElementById('tip_closed').style.display = 'block';
setTimeout(function () {
var content = domList.value;
content += "[ 正在重连... ]" + '\r\n';
domList.innerHTML = content;
fn_ConnectWebSocket();
}, 3000); // 3 秒后重连
};
}
// 检查连接状态
function fn_IsWebSocketConnected() {
if (webSocket.readyState === WebSocket.OPEN) {
document.getElementById('tip_closed').style.display = 'none';
return true;
} else {
document.getElementById('tip_closed').style.display = 'block';
return false;
}
}
// 发送内容
function fn_send() {
if (fn_IsWebSocketConnected()) {
var message = document.getElementById('msg').value;
document.getElementById('tip_required').style.display = message ? 'none' : 'block';
if (message) {
webSocket.send(JSON.stringify({
msg: message
}));
}
}
}
// 断开连接
function fn_disconnect() {
if (fn_IsWebSocketConnected()) {
// 部分浏览器调用 close() 方法关闭 WebSocket 时不支持传参
// webSocket.close(001, "Reason");
webSocket.close();
}
}
// 执行连接
fn_ConnectWebSocket();
</script>
</body>
</html>
【在线示例】
https://xoyozo.net/Demo/JsNetWebSocket
【其它】
-
服务端以 IIS 作为 Web 服务器,那么需要安装 WebSocket 协议
-
一个连接对应一个客户端(即 JS 中的 WebSocket 对象),注意与会话 Session 的区别
-
在实际项目中使用应考虑兼容性问题
-
程序设计应避免 XSS、CSRF 等安全隐患
-
参考文档:https://learn.microsoft.com/zh-cn/aspnet/core/fundamentals/websockets
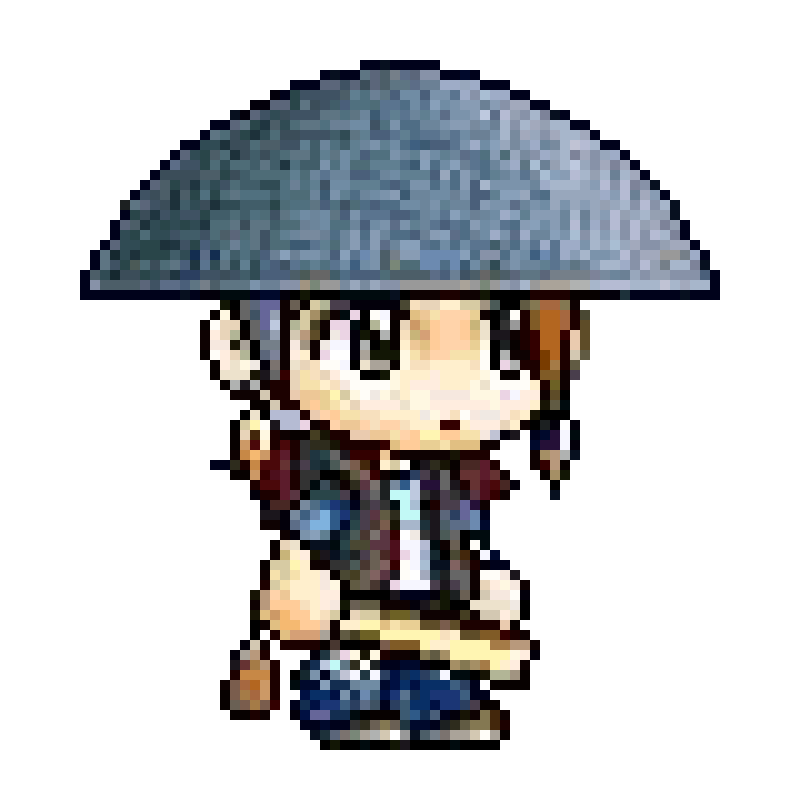
ChatGPT:
当你从 <textarea>
中获取到的数据中包含换行符时,如果直接在 Vue 模板中使用插值表达式 {{}}
显示,是无法保持换行的。因为在 HTML 中,多个连续的空格和换行符都会被视为一个空格,因此如果直接将 \n
显示在模板中,会被渲染成一个空格。
解决这个问题的方法之一是使用 CSS 样式来控制换行的显示。可以为显示数据的元素添加 white-space: pre-wrap;
样式,该样式可以保留换行符并自动换行。
例如,假设你的数据保存在 text
变量中,可以这样在模板中使用:
<pre style="white-space: pre-wrap;">{{ text }}</pre>
在上面的例子中,使用 <pre>
元素来包裹要显示的数据,并添加 white-space: pre-wrap;
样式,这样数据中的换行符就能被保留并自动换行了。
另外,如果你在 Vue 组件中使用了 v-html
指令来显示数据,也可以在数据中使用 <br>
标签来手动添加换行符,然后使用 v-html
来渲染数据。但是,这种方法需要注意 XSS 攻击的问题,因为 v-html
可以渲染任意的 HTML 标签和内容,如果数据中包含恶意代码,很容易被攻击者利用。
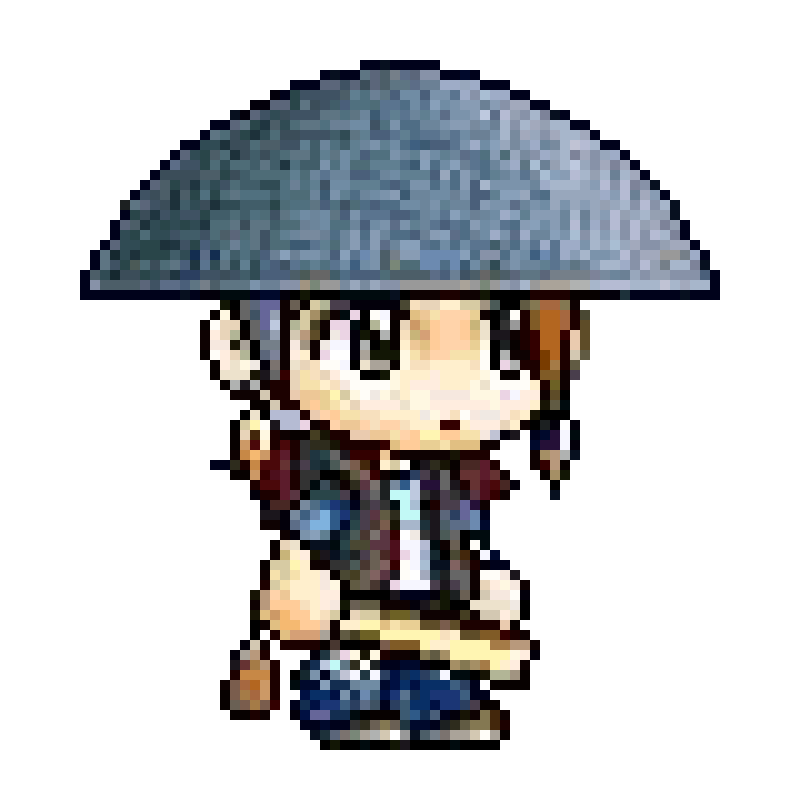
本文记录于 2021 年 9 月。
升级前 | 期望(最新正式版) | 最终选择 | |
操作系统 | CentOS 6.5 | Alibaba Cloud Linux 3 | Alibaba Cloud Linux 3 |
管理面板 | lnmp | 宝塔面板 Linux 版 7.7.0 | 宝塔面板 Linux 版 7.7.0 |
Web 服务 | nginx 1.6 | nginx 1.21 | nginx 1.21 |
脚本语言 | PHP 5.6 | PHP 8.0 | PHP 7.4 |
数据库 | RDS MySQL 5.6 | RDS MySQL 8.0 | RDS MySQL 5.6 |
论坛程序 | Discuz! X3.2 GBK | Discuz! X3.5 UTF-8(即将发布) | Discuz! X3.4 GBK |
版本选择原因:
Alibaba Cloud Linux 完全兼容 CentOS,相比于 CentOS 较短的生命周期,Alibaba Cloud Linux 3 将于 2029 年 4 月 30 日结束生命周期。
Discuz! X3.4 不支持 PHP 8.0,安装时即报错,打开页面时一片空白。
MySQL 8.0 和阿里云 RDS 的 MySQL 7.5 不支持 MyISAM,而数据表 pre_common_member_grouppm 和 pre_forum_post 使用联合主键且自动递增字段不是第一主键,使用 InnoDB 引擎创建表时会报“1075 - Incorrect table definition; there can be only one auto column and it must be defined as a key”错误,而擅自更改主键次序会影响业务逻辑。因此,在必须选择阿里云 RDS 的情况下,只能选择 MySQL 5.6。(2023年8月注:查看如何更改为 InnoDB)
Discuz! X3.5 正式版尚未发布(截止发稿),即便发布,插件也可能不能得到及时更新。相比之下,X3.4 首个版本发布距今已有 4 年,相关第三方插件已经非常成熟。
完整升级步骤:
备份原网站程序、RDS 数据库;
购买新的 ECS、RDS,挂载磁盘,安装云监控;
迁移(或还原)数据库到新的 RDS;
安装宝塔面板并配置;
安装 nginx 及 PHP;
创建网站、配置 SSL、伪静态、防盗链、可写目录禁执行等(.conf);
配置 hosts;
上传原网站程序到新的站点目录下;
按 Discuz! X 升级文档升级 X3.2 至 X3.4;详情见下文 ↓;
配置 OSS、Redis、更新缓存等;
测试论坛基本功能是否正常;检查附件是否正常显示;全面检查控制台配置;
逐个开启插件并检查兼容性;
按二开备忘录逐个按需进行二开;
逐个修改调用论坛接口的项目及直接调用论坛数据库的项目;
调试 MAGAPP 接口;
尝试强制 https 访问;
将以上所有修改后的程序保留备份;发布升级公告并关闭论坛;重复以上步骤;修改域名解析;开启论坛;
配置 IP 封禁、定时器、日志、自动备份、配置其它 ECS 的 hosts 等;
查看搜索引擎中收录的地址,是否有无法访问的情况;
尝试将历史遗留的本地附件全部转移到 OSS;
Discuz! X 升级步骤及注意点:
升级前务必先修改 ./config/ 目录下的数据库/缓存连接信息,以防出现新站连接老库的情况;
按官方文档进行升级;
【问题】运行到 ./install/update.php?step=data&op=notification 时白屏。
【排查】尝试切换到 PHP 5.6 后成功(但该版本过于陈旧不能使用);尝试升级 CPU 和内存 PHP 7.4 上升级仍不成功。
【原因】DB::result_first() 方法不对 SQL 语句追加“limit 1”,而是 SELECT 所有记录后在 PHP 端取第一条数据;
【解决】打开文件 update.php,查找 elseif($_GET['op'] == 'notification'),该节点的功能是在表 home_notification 中查找 category <= 0 的数据并修复它,如果数据库中所有 category 都大于 0,直接注释其内部 if 代码段继续升级即可(或改为 if(false && ...))。
【问题】发布主题遇到错误:(1062) Duplicate entry '*' for key 'pid'
【原因】forum_post 中的 pid 不是自动增长的,而是由表 forum_post_tableid 中自动增长的 pid 生成的。如果生成的 pid 值已在 forum_post 表中存在,则会出现此错误。
【解决】迁移数据库时应关闭论坛,以防止 forum_post 表有新数据插入。
【问题】打开帖子页面 ./thread-***-1-1.html 显示 404 Not Found,而 ./forum.php?mod=viewthread&tid=*** 可以正常打开
【原因】未配置伪静态(可在宝塔面板中选择)
【问题】打开 UCenter 时报错:UCenter info: MySQL Query Error SQL:SELECT value FROM [Table]vars WHERE name='noteexists'
【解决】打开文件 ./uc_server/data/config.inc.php 配置数据库连接
【问题】打开登录 UCenter 后一片空白
【解决】将目录 ./uc_server/data/ 设为可写
需要将原来安装的插件文件移回 ./source/plugin/ 目录,并设置可写;
界面-表情管理,界面-编辑器设置-Discuz!代码
后续 Discuz! X3.4 R 小版本升级注意事项:
确认插件是否支持新版本(如短信通)
先创建一个新网站测试二开代码
保留 /config/、/data/、/uc_client/data/、/uc_server/data/、/source/plugin/,其它移入 old
上传文件
移回其它需要的文件,如:
-- 勋章/loading/logo/nv 等:/static/image/common/
-- 表情:/static/image/smiley/
-- 水印:/static/image/common/watermark.*
-- 风格:/template/default/style/t2/nv.png 等
-- 默认头像:/uc_server/images/noavatar_***.gif
-- 根目录 favicon.ico 等
-- 及其它非 DZ 文件
再次检查可写目录的写入权限和禁止运行 PHP 效果。
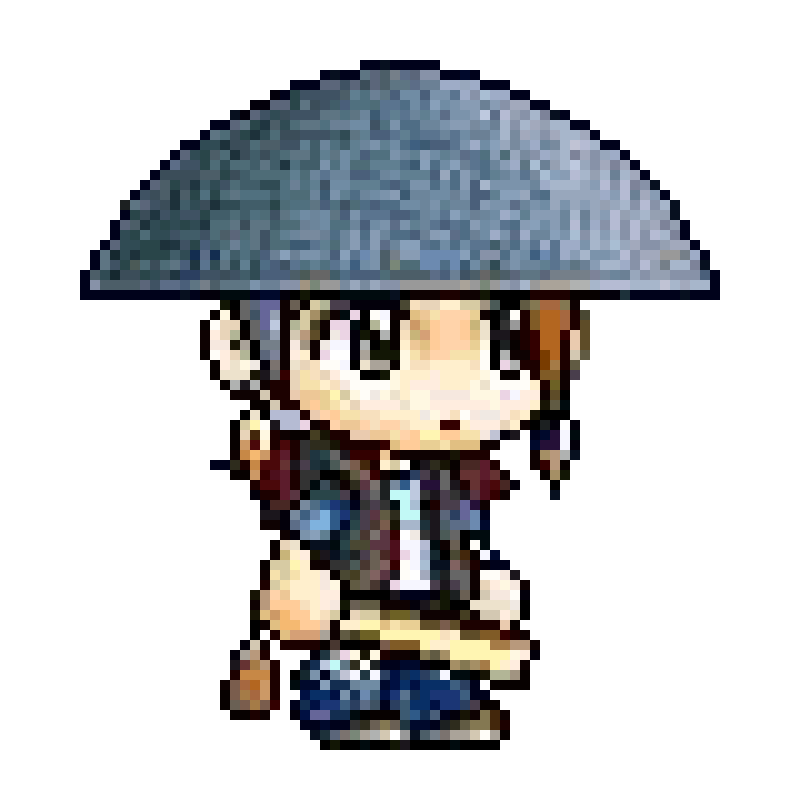
Unify Template 提供三种文件上传样式(如上图),“Plain File Input”和“File input”使用传统 <form /> 提交,“Advanced File input”调用组件实现。
ASP.NET Core 实现传统 <form /> 提交(以“Plain File Input”为例):
<form class="g-brd-around g-brd-gray-light-v4 g-pa-30 g-mb-30" id="form_avatar_upload" asp-action="AvatarUpload" enctype="multipart/form-data" method="post">
<!-- Plain File Input -->
<div class="form-group mb-0">
<p>Plain File Input</p>
<label class="u-file-attach-v2 g-color-gray-dark-v5 mb-0">
<input id="fileAttachment" name="Avatar" type="file" onchange="form_avatar_upload.submit()">
<i class="icon-cloud-upload g-font-size-16 g-pos-rel g-top-2 g-mr-5"></i>
<span class="js-value">Attach file</span>
</label>
</div>
<!-- End Plain File Input -->
</form>
public class AvatarUploadModel
{
public IFormFile Avatar { get; set; }
}
[HttpPost]
public IActionResult AvatarUpload(AvatarUploadModel Item)
{
return RedirectToAction("");
}
ASP.NET Core 调用 HSFileAttachment 组件实现:
<div class="form-group mb-20">
<label class="g-mb-10">上传作品文件(包)</label>
<input class="js-file-attachment_project" type="file" name="" id="file_project">
<div style="display: none;" id="file_project_tip">
<div class="u-file-attach-v3 g-mb-15" id="file_project_tip_content">
<h3 class="g-font-size-16 g-color-gray-dark-v2 mb-0">拖曳文件到此处或者 <span class="g-color-primary">浏览你的电脑</span></h3>
<p class="g-font-size-14 g-color-gray-light-v2 mb-0">
如果您的作品包含多个文件,请添加到压缩包再上传
</p>
<p class="g-font-size-14 g-color-gray-light-v2 mb-0">
支持的文件格式:
<code>zip</code>
<code>rar</code>
<code>7z</code>
<code>psd</code>
<code>ai</code>
<code>cdr</code>
<code>jpg</code>
<code>png</code>
<code>gif</code>
<code>tif</code>
<code>webp</code>
</p>
<p class="g-font-size-14 g-color-gray-light-v2 mb-0">文件(包)的大小不能超过 <span>@options.Value.MaxSizeOfSigleFile_MB</span> MB</p>
</div>
</div>
<small class="form-control-feedback">请上传作品文件</small>
</div>
<!-- JS Implementing Plugins -->
<script src="//cdn.***.com/unify/unify-v2.6.2/html/assets/vendor/jquery.filer/js/jquery.filer.min.js"></script>
<!-- JS Unify -->
<script src="//cdn.***.com/unify/unify-v2.6.2/html/assets/js/helpers/hs.focus-state.js"></script>
<script src="//cdn.***.com/unify/unify-v2.6.2/html/assets/js/components/hs.file-attachement.js"></script>
<!-- JS Plugins Init. -->
<script>
$(document).on('ready', function () {
// initialization of forms
$.HSCore.components.HSFileAttachment.init('.js-file-attachment_project', {
changeInput: $('#file_project_tip').html(),
uploadFile: {
url: url_UploadFile,
data: { type: 'project' },
success: function (data, element) {
console.log(data);
if (!data.success) {
var parent = element.find(".u-progress-bar-v1").parent();
element.find(".u-progress-bar-v1").fadeOut("slow", function () {
$("<div class=\"text-error g-px-10\"><i class=\"fa fa-minus-circle\"></i> Error</div>").hide().appendTo(parent).fadeIn("slow");
});
alert(data.err_msg);
} else {
var parent = element.find(".u-progress-bar-v1").parent();
element.find(".u-progress-bar-v1").fadeOut("slow", function () {
$("<div class=\"text-success g-px-10\"><i class=\"fa fa-check-circle\"></i> Success</div>").hide().appendTo(parent).fadeIn("slow");
});
$('#file_project_tip_content').slideUp();
upload_project_id = data.id;
}
}
}
});
$.HSCore.helpers.HSFocusState.init();
});
</script>
public IActionResult UploadFile([FromForm(Name = "[]")]IFormFile file, string type)
{
return Ok(new { id = 1 });
}
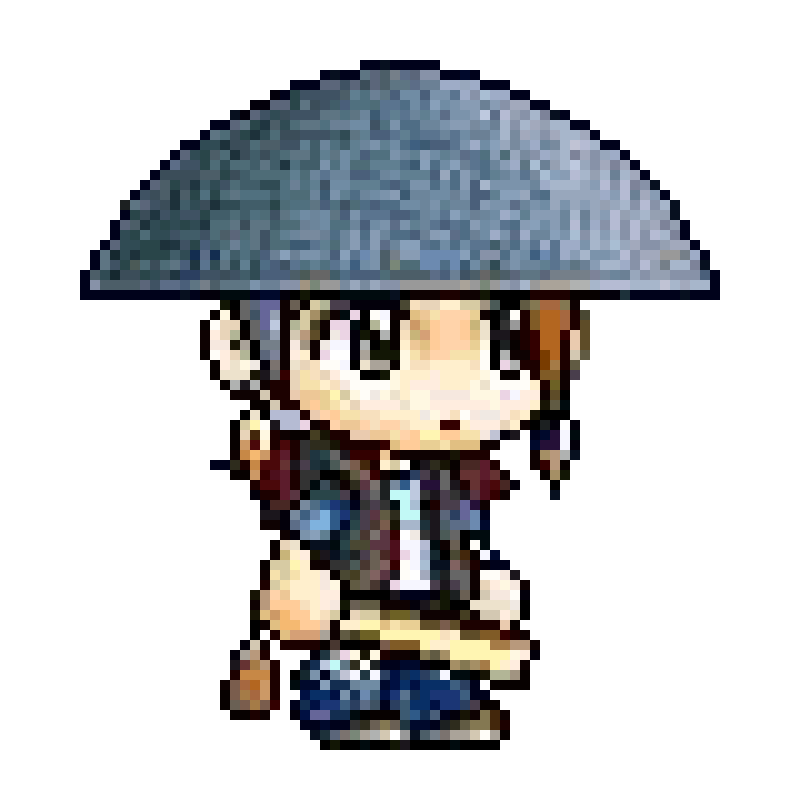
使用 JS 根据屏幕宽度来计算页面尺寸和字体大小并不是一个好办法。
rem 单位是跟随网页根元素的 font-size 改变而自动改变的,是一个相对的长度单位,非常适合在不同手机界面上自适应屏幕大小。
一般的手机浏览器的默认字体大小为 16px。即:
:root { font-size: 16px; } /* 在 HTML 网页中,:root 选择器相当于 html */
也就是说,如果我定义一个宽度为 1rem,那么它的实际宽度为 16px。反过来说,如果设计稿宽度为 1000px,那么相当于 1000÷16=62.5rem。因此,我们设置一个 62.5rem 的宽度,即可正常显示 1000px 的设计效果。
为了适应不同屏幕尺寸的手机,只需按比例换算更改 :root 的 font-size 即可。这个比例是由“窗口宽度 ÷ 设计稿宽度”得到的,姑且称它为“窗设比”。
以 iPhone X 逻辑宽度 375px 为例,设计稿宽度 1000px 时,窗设比为:0.375。:root 的 font-size 将会被重置为 16 * 0.375 = 6px。上面的 62.5rem 宽度将对应 iPhone X 宽度为 62.5 * 6 = 375px,正好是屏幕逻辑宽度。
根据这个思路,理论上,我们只需要使用 CSS 将 :root 的 font-size 设置为 1px,然后使用 JS 重置为与“窗设比”相乘的积(本例中将被重置为 0.375px)。这样,我们可以不通过换算,只需要更改单位,将设计稿中的 500px 直接写入到 CSS 为 500rem,即可实现在 iPhone X 上显示逻辑宽度为(500 * 0.375 =)187.5px,即设计稿中的 1/2 宽度对应到屏幕上 1/2 的宽度。
实际上,部分安卓手机(如华为某旗舰机)不支持 :root 的 font-size 小于 12px,其它一些浏览器也会出现小于 12px 的文字以 12px 显示。
为了避免在任何环节出现小于 12px 的 font-size 值,且不增加设计稿尺寸到 CSS 数值的换算难度,我们设计了以下思路:
约定“设样比(设计稿的 px 值与 CSS 的 rem 值的比)”为 100;
使用 JS 将 :root 的 font-size 重置为“设样比”与“窗设比(窗口宽度 ÷ 设计稿宽度)”的乘积;
计算 CSS 时的 rem 值只需从设计稿中获取 px 值再除以“设样比”100 即可。
这样做的另一个好处是,不用要求设计师必须按多少尺寸来设计,当然按主流尺寸来建议是可行的。
完整代码(jQuery 版):
<!DOCTYPE html>
<html lang="zh-cn">
<head>
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0" />
<title>Index</title>
<style>
body { margin: 0; text-align: center; }
#app { margin: 0 auto; background-color: #EEE; display: none; }
/* 长度计算方法:从设计稿获得长度(例如 160px),除以 px2rem 值(本例为 100),得到 1.6rem */
.whole { background-color: #D84C40; width: 10rem; }
.half { background-color: #3296FA; width: 5rem; }
.half2 { background-color: #3296FA; width: 5rem; margin-left: 5rem; }
</style>
</head>
<body>
<div id="app">
<div class="whole">100%</div>
<div class="half">50%</div>
<div class="half2">50%</div>
</div>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
// [函数] 重置尺寸
function fn_resize() {
// 设计稿宽度(px)
var designsWidth = 1000;
// 约定的“设计稿 px 值与 CSS rem 值的比”,为方便计算,一般无需改动
var px2rem = 100;
// 限制 #app 的最大宽度为设计稿宽度
$('#app').css('max-width', designsWidth).css('min-height', $(window).height());
// 重置 :root 的 font-size
var appWidth = Math.min($(window).width(), designsWidth);
$(':root').css('font-size', px2rem * appWidth / designsWidth);
$('#app').show();
}
// 页面加载完成后重置尺寸
$(fn_resize);
// 改变窗口大小时重置尺寸
$(window).resize(fn_resize);
</script>
</body>
</html>
完整代码(Vue2 版):
<!DOCTYPE html>
<html lang="zh-cn">
<head>
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0" />
<title>Index</title>
<style>
body { margin: 0; text-align: center; }
#app { margin: 0 auto; background-color: #EEE; }
/* 长度计算方法:从设计稿获得长度(例如 160px),除以 px2rem 值(本例为 100),得到 1.6rem */
.whole { background-color: #D84C40; width: 10rem; }
.half { background-color: #3296FA; width: 5rem; }
.half2 { background-color: #3296FA; width: 5rem; margin-left: 5rem; }
</style>
</head>
<body>
<div id="app" v-bind:style="{ maxWidth: designsWidth + 'px', minHeight: appMinHeight + 'px' }" style="display: none;" v-show="appShow">
<div class="whole">100%</div>
<div class="half">50%</div>
<div class="half2">50%</div>
<div>appWidth: {{ appWidth }}</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue@2"></script>
<script>
var app = new Vue({
el: '#app',
data: {
// 设计稿宽度(px)
designsWidth: 1000,
// 约定的“设计稿 px 值与 CSS rem 值的比”,为方便计算,一般无需改动
px2rem: 100,
// #app 的 width
appWidth: 0, // 勿改
appMinHeight: 0, // 勿改
appShow: false, // 勿改
},
mounted: function () {
// 页面加载完成后重置尺寸
this.fn_resize();
const that = this;
// 改变窗口大小时重置尺寸
window.onresize = () => {
return (() => {
console.log('RUN window.onresize()');
that.fn_resize();
})();
};
},
watch: {
// 侦听 appWidth 更改 root 的 font-size
appWidth: function () {
console.log('RUN watch: appWidth');
var root = document.getElementsByTagName("html")[0];
root.style.fontSize = (this.px2rem * this.appWidth / this.designsWidth) + 'px';
this.appShow = true;
}
},
methods: {
fn_resize: function () {
console.log('RUN methods: fn_resize()');
this.appWidth = Math.min(document.body.clientWidth, this.designsWidth);
this.appMinHeight = document.documentElement.clientHeight;
}
}
});
</script>
</body>
</html>
完整代码(Vue3 版):
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0" />
<title>Index</title>
<style>
body { margin: 0; text-align: center; }
#app > div { display: none; margin: 0 auto; background-color: #EEE; }
/* 长度计算方法:从设计稿获得长度(例如 160px),除以 px2rem 值(本例为 100),得到 1.6rem */
.whole { background-color: #D84C40; width: 10rem; }
.half { background-color: #3296FA; width: 5rem; }
.half2 { background-color: #3296FA; width: 5rem; margin-left: 5rem; }
</style>
</head>
<body>
<div id="app">
<div style="display: none;" v-bind:style="{ maxWidth: designsWidth + 'px', minHeight: appMinHeight + 'px', display: appShow ? 'block' : 'none' }">
<div class="whole">100%</div>
<div class="half">50%</div>
<div class="half2">50%</div>
<div>appWidth: {{ appWidth }}</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue@3"></script>
<script>
const app = Vue.createApp({
data: function () {
return {
// 设计稿宽度(px)
designsWidth: 1000,
// 约定的“设计稿 px 值与 CSS rem 值的比”,为方便计算,一般无需改动
px2rem: 100,
// #app 的 width
appWidth: 0, // 勿改
appMinHeight: 0, // 勿改
appShow: false, // 勿改
}
},
mounted: function () {
// 页面加载完成后重置尺寸
this.fn_resize();
const that = this;
// 改变窗口大小时重置尺寸
window.onresize = () => {
return (() => {
console.log('RUN window.onresize()');
that.fn_resize();
})();
};
},
watch: {
// 侦听 appWidth 更改 root 的 font-size
appWidth: function () {
console.log('RUN watch: appWidth');
var root = document.getElementsByTagName("html")[0];
root.style.fontSize = (this.px2rem * this.appWidth / this.designsWidth) + 'px';
this.appShow = true;
}
},
methods: {
fn_resize: function () {
console.log('RUN methods: fn_resize()');
this.appWidth = Math.min(document.body.clientWidth, this.designsWidth);
this.appMinHeight = document.documentElement.clientHeight;
}
}
});
const vm = app.mount('#app');
</script>
</body>
</html>
示例中 CSS 初始 :root 的 font-size 为 16px(一个较小值,防止页面加载时瞬间出现大号文字影响用户体验),经过 fn_resize 后,:root 的 font-size 被设置为(100 * 375 / 1000 =)37.5px(iPhone X 中),那么宽度为 1000px 的设计稿中的 500px 换算到 CSS 中为 5rem,也即 37.5 * 5 = 187.5px,就是 iPhone X 的屏幕宽度的一半。
示例中 id 为 app 的 div 是用来在 PC 浏览器中限制页面内容最大宽度的(类似微信公众号发布的文章),如果网页不需要在 PC 端显示,jQuery 版代码中的 $('#app').width() 可以用 $(window).width() 来代替。
这个 div#app 一般设计有背景色,用于在宽度超过设计稿的设备上显示时区别于 body 的背景。但是当网页内容不超过一屏时,div#app 高度小于窗口,示例中与 appMinHeight 相关的代码就是为了解决这个问题。
示例中 div#app 隐藏/显示相关代码用于解决在页面加载初期由于 font-size 值变化引起的一闪而过的排版错乱。
最后补充一点,如果改变窗口大小时涉及执行耗时的操作,为避免页面卡顿,可以参考这篇文章添加函数防抖:https://xoyozo.net/Blog/Details/js-function-anti-shake
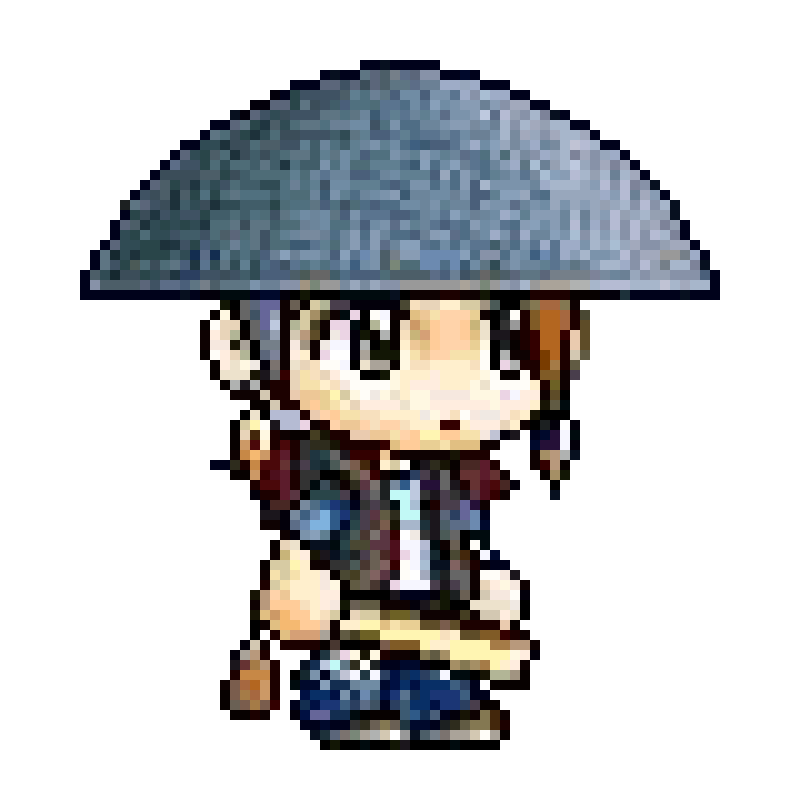
Nuget 安装:X.PagedList.Mvc.Core
控制器:
using X.PagedList;
public IActionResult Index(int page = 1)
{
……
return View(q.ToPagedList(page, size));
}
视图:
@using X.PagedList
@using X.PagedList.Mvc.Core
@model IPagedList<xxx>
@Html.PagedListPager(Model, page => Url.Action("Index", new { page }))
自定义(options 默认值):
@Html.PagedListPager(
Model,
page => Url.Action("Index", new { page }),
new X.PagedList.Mvc.Common.PagedListRenderOptionsBase
{
HtmlEncoder = HtmlEncoder.get_Default(),
DisplayLinkToFirstPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToLastPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToPreviousPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToNextPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToIndividualPages = true,
DisplayPageCountAndCurrentLocation = false, // 显示总页数和当前页码
MaximumPageNumbersToDisplay = 10, // 最多显示页码数
DisplayEllipsesWhenNotShowingAllPageNumbers = true,
EllipsesFormat = "…",
LinkToFirstPageFormat = "<<",
LinkToPreviousPageFormat = "<",
LinkToIndividualPageFormat = "{0}",
LinkToNextPageFormat = ">",
LinkToLastPageFormat = ">>",
PageCountAndCurrentLocationFormat = "Page {0} of {1}.",
ItemSliceAndTotalFormat = "Showing items {0} through {1} of {2}.",
FunctionToDisplayEachPageNumber = null,
ClassToApplyToFirstListItemInPager = null,
ClassToApplyToLastListItemInPager = null,
ContainerDivClasses = new string[1]
{
"pagination-container"
},
UlElementClasses = new string[1]
{
"pagination"
},
LiElementClasses = Enumerable.Empty<string>(),
PageClasses = Enumerable.Empty<string>(),
UlElementattributes = null,
ActiveLiElementClass = "active",
EllipsesElementClass = "PagedList-ellipses",
PreviousElementClass = "PagedList-skipToPrevious",
NextElementClass = "PagedList-skipToNext",
})
保留地址栏参数:
@{
string query = Context.Request.QueryString.Value;
}
@Html.PagedListPager(Model, page => Regex.IsMatch(query, @"[?&]page=\d+")
? Regex.Replace(query, @"([?&])page=\d+", $"$1page={page}")
: (query.StartsWith("?") ? $"{query}&page={page}" : $"{query}?page={page}"),
new X.PagedList.Web.Common.PagedListRenderOptionsBase
{
DisplayPageCountAndCurrentLocation = true,
MaximumPageNumbersToDisplay = 5,
})
这里从查询字符串中判断并替换 page 值,如果有更简单的方法敬请告知。比如 Webdiyer 的分页组件会自动携带所有参数。
更多使用方式参官方文档:https://github.com/dncuug/X.PagedList
附应用于 Unify Template(一款基于 Bootstrap 的 HTML 模板)中的配置:
<style>
.u-pagination-v1-1--active .u-pagination-v1-1 { color: #fff; border-color: #72c02c; }
.PagedList-pageCountAndLocation { float: right !important; }
</style>
@{
string query = Context.Request.QueryString.Value;
}
@Html.PagedListPager(Model, page => Regex.IsMatch(query, @"[?&]page=\d+")
? Regex.Replace(query, @"([?&])page=\d+", $"$1page={page}")
: (query.StartsWith("?") ? $"{query}&page={page}" : $"{query}?page={page}"),
new X.PagedList.Web.Common.PagedListRenderOptionsBase
{
DisplayPageCountAndCurrentLocation = true,
MaximumPageNumbersToDisplay = 5,
UlElementClasses = new string[] { "list-inline" },
LiElementClasses = new string[] { "list-inline-item" },
PageClasses = new string[] { "u-pagination-v1__item", "u-pagination-v1-1", "g-pa-7-14" },
ActiveLiElementClass = "u-pagination-v1-1--active",
EllipsesElementClass = "g-pa-7-14",
})
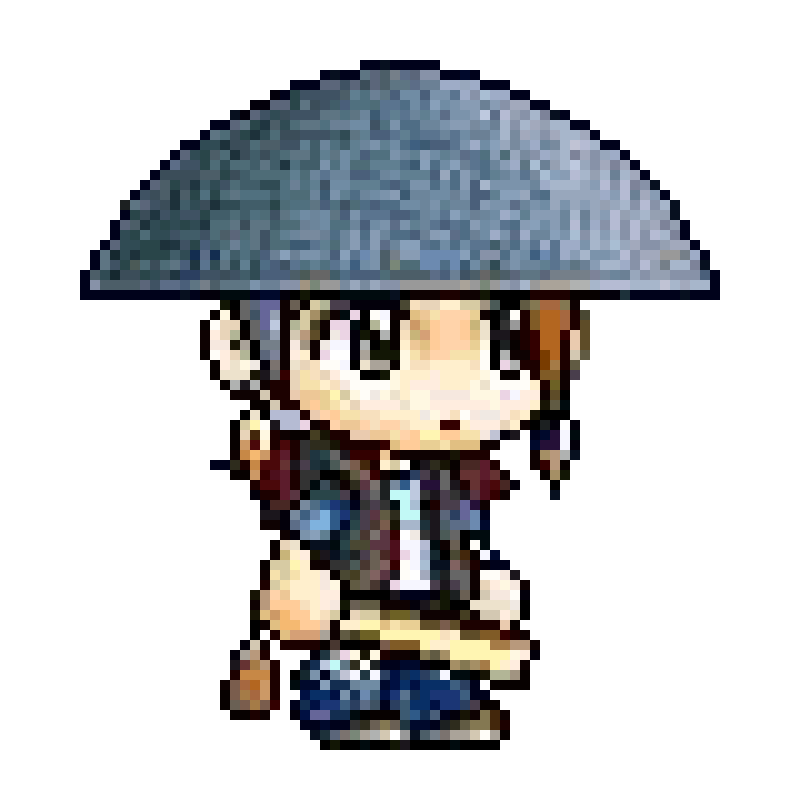
安装 Nuget 包:
项目根目录创建绑定配置文件:bundleconfig.json
示例:
[
{
"outputFileName": "wwwroot/css/site.min.css",
"inputFiles": [
"wwwroot/css/site.css",
"wwwroot/css/custom.css"
]
},
{
"outputFileName": "wwwroot/js/site.min.js",
"inputFiles": [
"wwwroot/js/site.js"
],
"minify": {
"enabled": true,
"renameLocals": true
},
"sourceMap": false
}
]
配置文件中所有路径都相对于项目根目录(而非静态文件根目录 wwwroot),因此配置的路径都要以“wwwroot”开头。
outputFileName 是压缩合并后的文件,inputFiles 是被压缩合并的原始文件集合。
对于 js 配置部分,minify.enabled 配置是否缩小,renameLocals 配置是否修改变量名,sourceMap 配置是否生成 map 映射文件。
引用示例:
<link rel="stylesheet" href="~/css/site.min.css" asp-append-version="true" />
asp-append-version 表示是否在引用路径添加版本参数,可实现在文件有修改时及时在客户端浏览器中生效。
* 注意:有时候“生成”不一定生效,“重新生成”肯定会生效。
更多高级用法请参考官方文档。
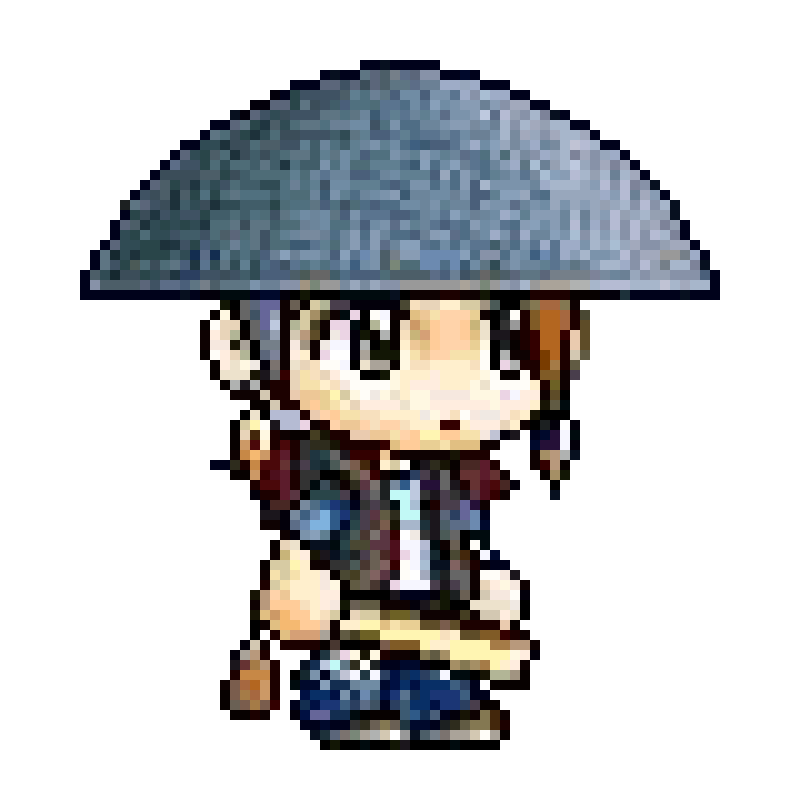
完整操作步骤如下:
安装 NuGet 包:
Microsoft.AspNet.Web.Optimization
打开
Views
目录(如果是应用于区域,则为区域的Views
目录)中的 web.config,在<namespaces />
节点中添加<add namespace="System.Web.Optimization" />
在
App_Start
目录中创建类文件BundleConfig.cs
,更改其命名空间为应用程序的默认命名空间(即移除.App_Start
),创建方法:public static void RegisterBundles(BundleCollection bundles) { bundles.Add(new StyleBundle("~/虚拟路径(不能有点)").Include("~/CSS文件路径")); bundles.Add(new ScriptBundle("~/虚拟路径(不能有点)").Include("~/JS文件路径")); }
虚拟路径应避免与页面访问路径相同。Include 可包含多个文件,效果是合并输出,注意引用的顺序。
打开
Global.asax
,在Application_Start()
事件中添加代码BundleTable.EnableOptimizations = true; // 该设置使在开发模式中实现压缩代码,不设置则仅在发布后压缩代码 BundleConfig.RegisterBundles(BundleTable.Bundles);
视图页面中引用样式表或脚本
@Styles.Render("~/CSS虚拟路径") @Scripts.Render("~/JS虚拟路径")
使用 Render 的好处是,ASP.NET 会自动给引用地址加上参数,可在更改脚本或样式表内容后更改这些参数使浏览器缓存立即失效。
如果你的项目中已经安装并使用 Bundle
,那么只需要参考第 4 步,将 BundleTable
的 EnableOptimixations
设为 true
。
以下是一些常见异常的解决方法:
The name 'Styles' does not exist in the current context
The name 'Scripts' does not exist in the current context
解决:参步骤 2。
引用的样式表或脚本不存在(报 404
错误)
解决:步骤 3 中的虚拟路径不规范。
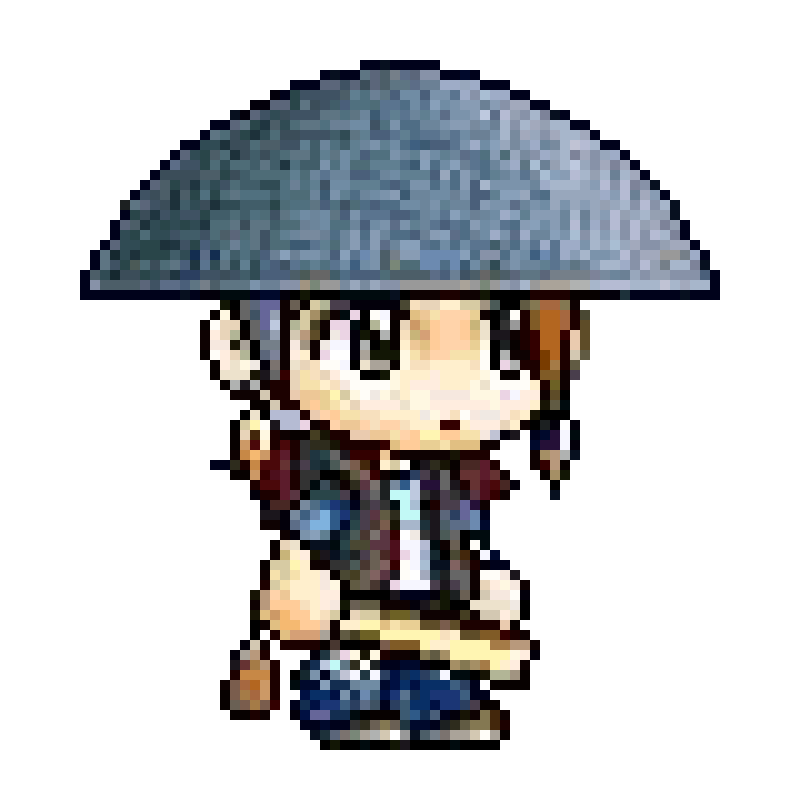
百度地图 | 天地图 | |
初始化地图 | var map = new BMap.Map("allmap"); | var map = new T.Map("allmap"); |
将覆盖物添加到地图 | addOverlay(overlay: Overlay) | addOverLay(overlay:OverLay) |
从 Map 的 click 事件中获取坐标点 | map.addEventListener("click", function (e) { Point = e.point; } | map.addEventListener("click", function (e) { LngLat = e.lnglat; } |
坐标点 | new BMap.Point(lng: Number, lat: Number) | new T.LngLat(lng: Numbe, lat: Number) |
像素点 | new BMap.Pixel(x: Number, y: Number) | new T.Point(x: Number, y: Number) |
文本标注 |
|
|
设置文本标注样式 | setStyle(styles: Object) | 每个样式使用单独的 set 方法实现 |
图像标注 |
|
|
为图像标注添加文本标注 | Marker.setLabel(label: Label) | 无。 可借用 title(鼠标掠过显示)属性来实现,并通过 Marker.options.title 来获取值 |
从图像标注获取坐标点 | Point = Marker.getPosition(); | LngLat = Marker.getLngLat(); |
绘制折线 |
|
|
绘制多边形 |
|
|
设置多边形的点数组 | Polygon.setPath(points: Array<Point>) | Polyline.setLngLats(lnglats: Array<LngLat>) |
设置地图视野 | Map.setViewport(view: Array<Point> | Viewport, viewportOptions: ViewportOptions) | Map.setViewport(view: Array<LngLat>) |
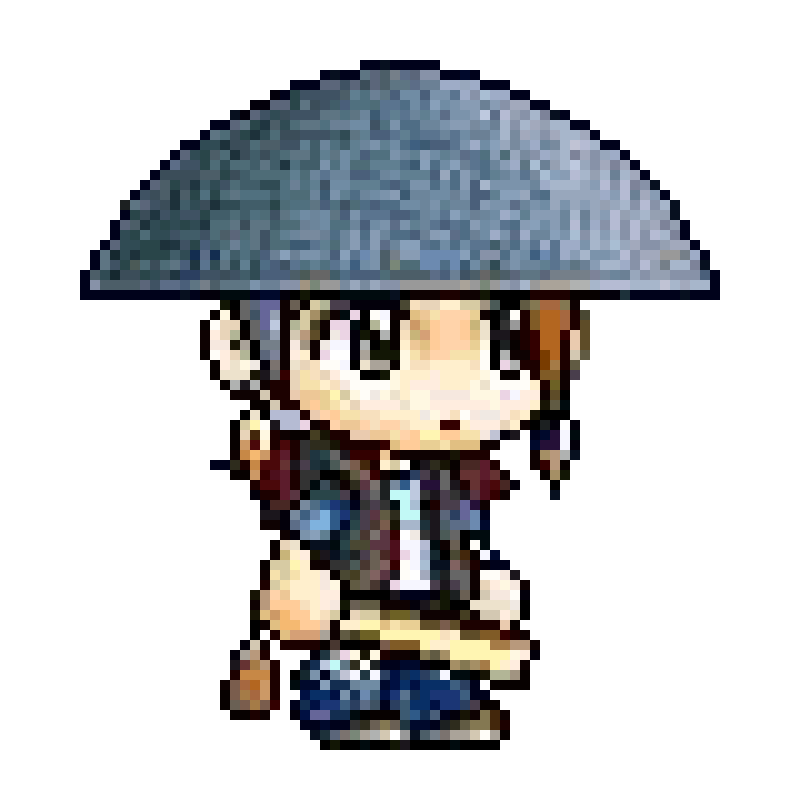