推荐使用 SignalR 来平替 WebSocket,参考教程。
【服务端(.NET)】
一、创建 WebSocketHandler 类,用于处理客户端发送过来的消息,并分发到其它客户端
public class WebSocketHandler
{
private static List<WebSocket> connectedClients = [];
public static async Task Handle(WebSocket webSocket)
{
connectedClients.Add(webSocket);
byte[] buffer = new byte[1024];
WebSocketReceiveResult result = await webSocket.ReceiveAsync(new ArraySegment<byte>(buffer), CancellationToken.None);
while (!result.CloseStatus.HasValue)
{
string message = Encoding.UTF8.GetString(buffer, 0, result.Count);
Console.WriteLine($"Received message: {message}");
// 处理接收到的消息,例如广播给其他客户端
await BroadcastMessage(message, webSocket);
result = await webSocket.ReceiveAsync(new ArraySegment<byte>(buffer), CancellationToken.None);
}
connectedClients.Remove(webSocket);
await webSocket.CloseAsync(result.CloseStatus.Value, result.CloseStatusDescription, CancellationToken.None);
}
private static async Task BroadcastMessage(string message, WebSocket sender)
{
foreach (var client in connectedClients)
{
if (client != sender && client.State == WebSocketState.Open)
{
await client.SendAsync(Encoding.UTF8.GetBytes(message), WebSocketMessageType.Text, true, CancellationToken.None);
}
}
}
}
二、在 Program.cs 或 Startup.cs 中插入:
// 添加 WebSocket 路由
app.UseWebSockets();
app.Use(async (context, next) =>
{
if (context.Request.Path == "/chat")
{
if (context.WebSockets.IsWebSocketRequest)
{
WebSocket webSocket = await context.WebSockets.AcceptWebSocketAsync();
await WebSocketHandler.Handle(webSocket);
}
else
{
context.Response.StatusCode = 400;
}
}
else
{
await next();
}
});
这里的路由路径可以更改,此处将会创建一个 WebSocket 连接,并将其传递给 WebSocketHandler.Handle 方法进行处理。
也可以用控制器来接收 WebSocket 消息。
【客户端(JS)】
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0" />
<title>WebSocket 示例(JS + ASP.NET)</title>
<style>
#list { width: 100%; max-width: 500px; }
.tip { color: red; }
</style>
</head>
<body>
<h2>WebSocket 示例(JS + ASP.NET)</h2>
<textarea id="list" readonly rows="20"></textarea>
<div>
<input type="text" id="msg" />
<button onclick="fn_send()">发送</button>
<button onclick="fn_disconnect()">断开</button>
</div>
<p id="tip_required">请输入要发送的内容</p>
<p id="tip_closed">WebSocket 连接未建立</p>
<script>
var wsUrl = "wss://" + window.location.hostname + ":" + window.location.port + "/chat";
var webSocket;
var domList = document.getElementById('list');
// 初始化 WebSocket 并连接
function fn_ConnectWebSocket() {
webSocket = new WebSocket(wsUrl);
// 向服务端发送连接请求
webSocket.onopen = function (event) {
var content = domList.value;
content += "[ WebSocket 连接已建立 ]" + '\r\n';
domList.innerHTML = content;
document.getElementById('tip_closed').style.display = 'none';
webSocket.send(JSON.stringify({
msg: 'Hello'
}));
};
// 接收服务端发送的消息
webSocket.onmessage = function (event) {
if (event.data) {
var content = domList.value;
content += event.data + '\r\n';
domList.innerHTML = content;
domList.scrollTop = domList.scrollHeight;
}
};
// 各种情况导致的连接关闭或失败
webSocket.onclose = function (event) {
var content = domList.value;
content += "[ WebSocket 连接已关闭,3 秒后自动重连 ]" + '\r\n';
domList.innerHTML = content;
document.getElementById('tip_closed').style.display = 'block';
setTimeout(function () {
var content = domList.value;
content += "[ 正在重连... ]" + '\r\n';
domList.innerHTML = content;
fn_ConnectWebSocket();
}, 3000); // 3 秒后重连
};
}
// 检查连接状态
function fn_IsWebSocketConnected() {
if (webSocket.readyState === WebSocket.OPEN) {
document.getElementById('tip_closed').style.display = 'none';
return true;
} else {
document.getElementById('tip_closed').style.display = 'block';
return false;
}
}
// 发送内容
function fn_send() {
if (fn_IsWebSocketConnected()) {
var message = document.getElementById('msg').value;
document.getElementById('tip_required').style.display = message ? 'none' : 'block';
if (message) {
webSocket.send(JSON.stringify({
msg: message
}));
}
}
}
// 断开连接
function fn_disconnect() {
if (fn_IsWebSocketConnected()) {
// 部分浏览器调用 close() 方法关闭 WebSocket 时不支持传参
// webSocket.close(001, "Reason");
webSocket.close();
}
}
// 执行连接
fn_ConnectWebSocket();
</script>
</body>
</html>
【在线示例】
https://xoyozo.net/Demo/JsNetWebSocket
【其它】
-
服务端以 IIS 作为 Web 服务器,那么需要安装 WebSocket 协议
-
一个连接对应一个客户端(即 JS 中的 WebSocket 对象),注意与会话 Session 的区别
-
在实际项目中使用应考虑兼容性问题
-
程序设计应避免 XSS、CSRF 等安全隐患
-
参考文档:https://learn.microsoft.com/zh-cn/aspnet/core/fundamentals/websockets
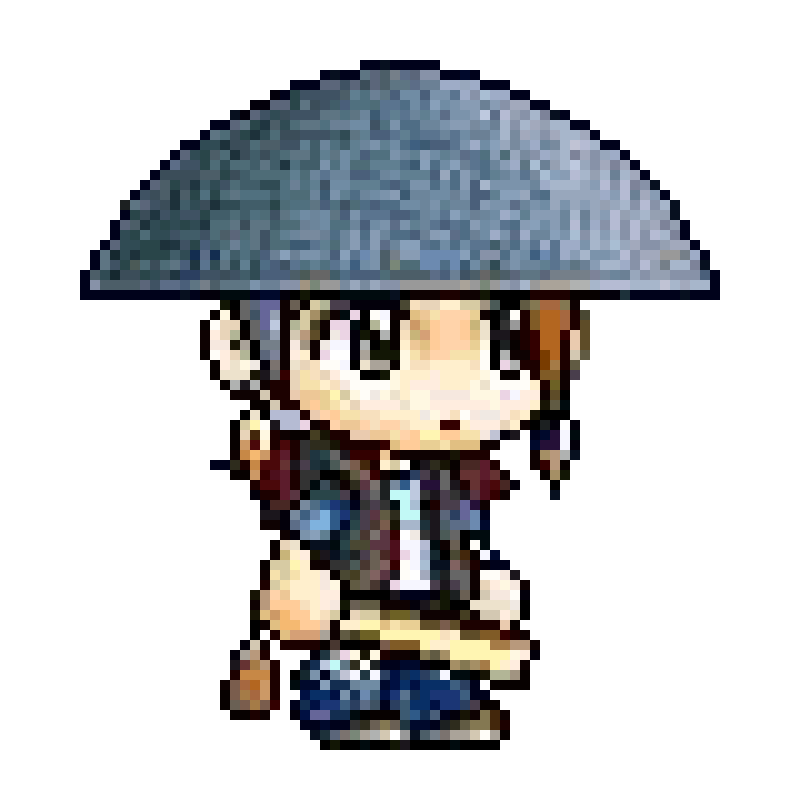
ChatGPT:
当你从 <textarea>
中获取到的数据中包含换行符时,如果直接在 Vue 模板中使用插值表达式 {{}}
显示,是无法保持换行的。因为在 HTML 中,多个连续的空格和换行符都会被视为一个空格,因此如果直接将 \n
显示在模板中,会被渲染成一个空格。
解决这个问题的方法之一是使用 CSS 样式来控制换行的显示。可以为显示数据的元素添加 white-space: pre-wrap;
样式,该样式可以保留换行符并自动换行。
例如,假设你的数据保存在 text
变量中,可以这样在模板中使用:
<pre style="white-space: pre-wrap;">{{ text }}</pre>
在上面的例子中,使用 <pre>
元素来包裹要显示的数据,并添加 white-space: pre-wrap;
样式,这样数据中的换行符就能被保留并自动换行了。
另外,如果你在 Vue 组件中使用了 v-html
指令来显示数据,也可以在数据中使用 <br>
标签来手动添加换行符,然后使用 v-html
来渲染数据。但是,这种方法需要注意 XSS 攻击的问题,因为 v-html
可以渲染任意的 HTML 标签和内容,如果数据中包含恶意代码,很容易被攻击者利用。
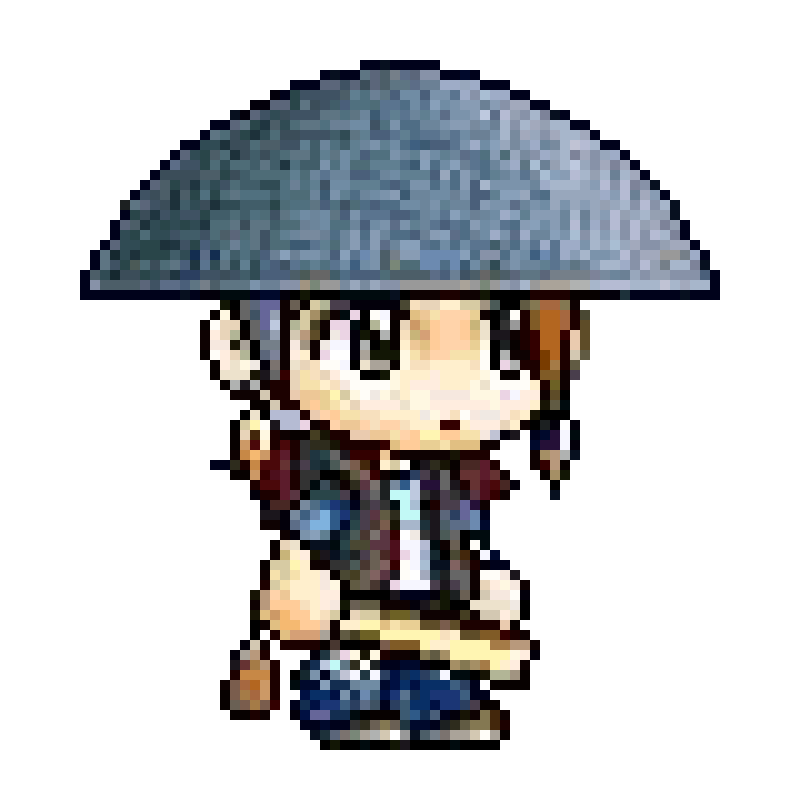
[
{
"outputFileName": "wwwroot/js/Admin/Blog/List.js",
"inputFiles": [
"Areas/Admin/Views/Blog/List.cshtml.js"
]
}
]
JS 源文件在视图目录,独立于 .cshtml 文件,又折叠于 .cshtml 文件。
输出目录在 wwwroot 中,若文件名不以“.min.js”结尾,那么会自动生成“.js”和“.min.js”两个文件,分别在开发模式和生产模式的视图中引用。
缺点:只更改 JS 内容,执行“生成解决方案”项目不会重新编译更新 wwwroot 中的 .js 和 .min.js 文件,这时使用“重新生成解决方案”可以解决这个问题。
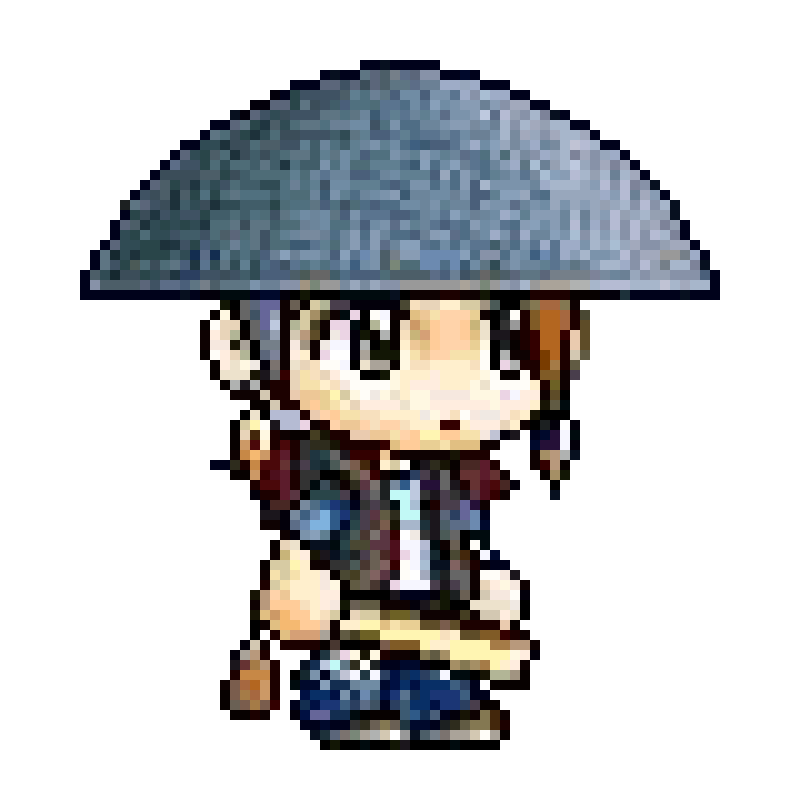
m① R②
x① R②
① 表示屏幕尺寸,一般有 15、17 等
② 表示第几代,对应 CPU 和显卡的不同,数字越大越新
相同 ① 与 ② 的情况下仍有细分款式,对应内存、硬盘、屏幕分辨率刷新率等不同
x 系列是全新的系列,是 m 系列的更新款(m 系列不再更新),相比 m 系列提升了散热性能
屏幕尺寸 | 型号 | 处理器 | 显卡 | 发布时间 |
14.0 英寸 | x14 R1 | 12 代 i7 | 30 系 | 2022年1月 |
15.6 英寸 | m15 R4 | 10 代 i7/i9 | 30 系 | |
m15 R5 | 锐龙 R7-5800H | 30 系 | ||
m15 R6 | 11 代 i7 | 30 系 | ||
m15 R7 | 12 代 i7 | 30 系 | 2022年2月 | |
x15 R1 | 11 代 i7/i9 | 30 系 | ||
x15 R2 | 12 代 i9 | 30 系 | 2022年2月 | |
16.0 英寸 | m16 | 13 代 i7/i9 | 40 系 | 2023年2月 |
x16 | 13 代 HK | 40 系 | 2023年2月 | |
17.3 英寸 | m17 R3 | 10 代 i7 | 20 系 | |
m17 R4 | 10 代 i7 | 30 系 | ||
m17 R5 | 锐龙 R7/R9 | 30 系 | 2022年3月 | |
x17 R1 | 11 代 i7/i9 | 30 系 | ||
x17 R2 | 12 代 i9K | 30 系 | 2022年2月 | |
18英寸 | m18 | 13 代 i9 | 40 系 | 2023年2月 |
x15 是板载内存,x17 是卡槽内存
x 系列屏幕有 1K165Hz / 1K360Hz / 2K240Hz(15寸独有)/ 4K120Hz(17寸独有)
x 系列有 2 个 M.2 硬盘位,没有 2.5 寸硬盘位
Area-51m R(数字):可拆 CPU
Area-51m R2:10 代 i7,20 系显卡
以上规则整理于 2021 年 7 月,随着时间的推移,以上信息将逐渐失效。
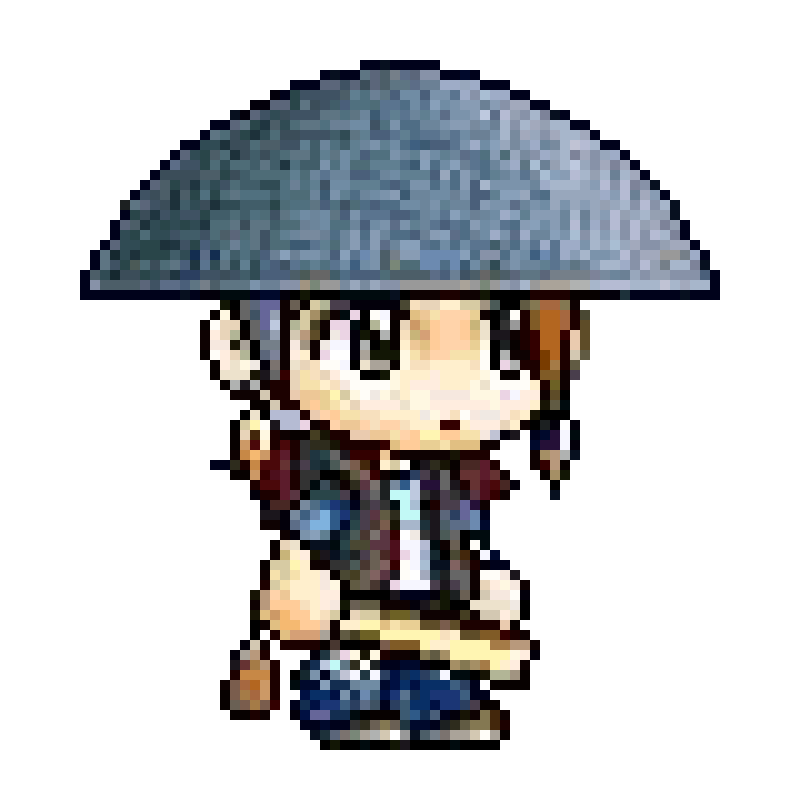
fail: Microsoft.AspNetCore.Mvc.ViewFeatures.ViewResultExecutor[3]
The view 'Index' was not found. Searched locations: /Areas/AAA/Views/XXX/Index.cshtml, /Areas/AAA/Views/Shared/Index.cshtml, /Views/Shared/Index.cshtml
fail: Microsoft.AspNetCore.Diagnostics.ExceptionHandlerMiddleware[1]
An unhandled exception has occurred while executing the request.
System.InvalidOperationException: The view 'Index' was not found. The following locations were searched:
/Areas/AAA/Views/XXX/Index.cshtml
/Areas/AAA/Views/Shared/Index.cshtml
/Views/Shared/Index.cshtml
开发环境一切正常,发布到服务器上出现上述错误,找不到视图文件,可视图文件明明就存在。
尝试新建一个文件,将 Index.cshtml 文件的内容拷贝到新文件中保存,删除原文件,将新文件改名为 Index.cshtml,发布,OK。
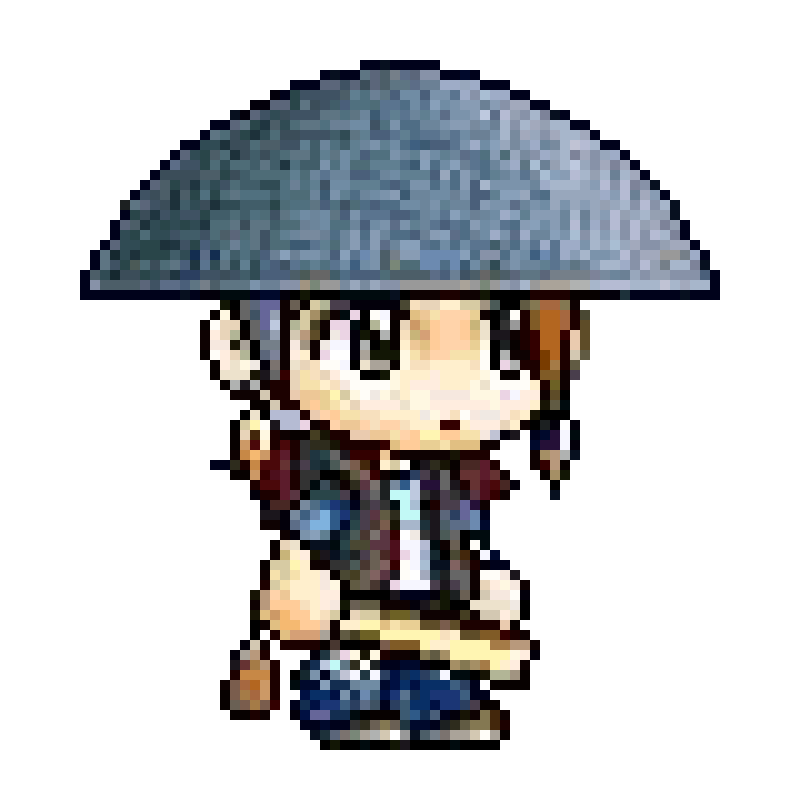
纯真 IP 数据库官方下载地址:http://www.cz88.net/
下载安装纯真 IP,将 qqwry.dat 文件复制到项目下。(本文以放在 /App_Data/ 目录下为例)
在 qqwry.dat 上右键打开属性窗口,将“复制到输出目录”切换到“始终复制”或“如果较新则复制”。
通过 nuget 安装 QQWry,喜欢依赖注入方式的可以选择 QQWry.DependencyInjectio。
var config = new QQWryOptions
{
DbPath = dbPath ?? (AppContext.BaseDirectory + @"App_Data\qqwry.dat")
};
var ipSearch = new QQWryIpSearch(config);
var ipl = ipSearch.GetIpLocation(ip);
return new IpLocation
{
Ip = ipl.Ip,
Country = ipl.Country?.Replace("CZ88.NET", "").Trim(),
Area = ipl.Area?.Replace("CZ88.NET", "").Trim(),
};
github 开源地址:https://github.com/JadynWong/IP_qqwry
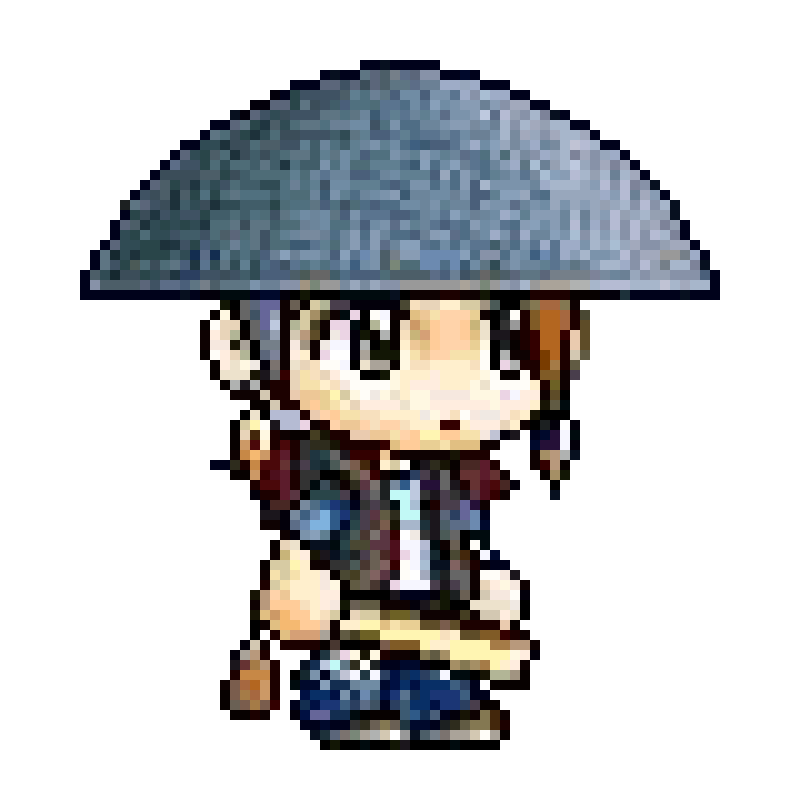
ASP.NET MVC 使用 Authorize 过滤器验证用户登录。Authorize 过滤器首先运行在任何其它过滤器或动作方法之前,主要用来做登录验证或者权限验证。
示例:使用 Authorize 过滤器实现简单的用户登录验证。
1、创建登录控制器 LoginController
/// <summary>
/// 登录控制器
/// </summary>
[AllowAnonymous]
public class LoginController : Controller
{
/// <summary>
/// 登录页面
/// </summary>
public ActionResult Index()
{
return View();
}
/// <summary>
/// 登录
/// </summary>
[HttpPost]
public ActionResult Login(string loginName, string loginPwd)
{
if (loginName == "admin" && loginPwd == "123456")
{
// 登录成功
Session["LoginName"] = loginName;
return RedirectToAction("Index", "Home");
}
else
{
// 登录失败
return RedirectToAction("Index", "Login");
}
}
/// <summary>
/// 注销
/// </summary>
public ActionResult Logout()
{
Session.Abandon();
return RedirectToAction("Index", "Login");
}
}
注意:在登录控制器 LoginController 上添加 AllowAnonymous 特性,该特性用于标记在授权期间要跳过 AuthorizeAttribute 的控制器和操作。
2、创建登录页面
@{
ViewBag.Title = "登录页面";
Layout = null;
}
<h2>登录页面</h2>
<form action='@Url.Action("Login","Login")' id="form1" method="post">
用户:<input type="text" name="loginName" /><br />
密码:<input type="password" name="loginPwd" /><br />
<input type="submit" value="登录">
</form>
效果图:
3、创建主页控制器 LoginController
public class HomeController : Controller
{
public ActionResult Index()
{
// 获取当前登录用户
string loginName = Session["LoginName"].ToString();
ViewBag.Message = "当前登录用户:" + loginName;
return View();
}
}
4、创建主页页面
@{
ViewBag.Title = "Index";
Layout = null;
}
<h2>Index</h2>
<h3>@ViewBag.Message</h3>
<a href="@Url.Action("Logout","Login")">注销</a>
效果图:
5、创建授权过滤器 LoginAuthorizeAttribute 类
创建 Filter 目录,在该目录下创建授权过滤器 LoginAuthorizeAttribute 类,继承 AuthorizeAttribute。
using System.Web.Mvc;
namespace MvcApp.Filter
{
/// <summary>
/// 授权过滤器
/// </summary>
public class LoginAuthorizeAttribute : AuthorizeAttribute
{
public override void OnAuthorization(AuthorizationContext filterContext)
{
// 判断是否跳过授权过滤器
if (filterContext.ActionDescriptor.IsDefined(typeof(AllowAnonymousAttribute), true)
|| filterContext.ActionDescriptor.ControllerDescriptor.IsDefined(typeof(AllowAnonymousAttribute), true))
{
return;
}
// 判断登录情况
if (filterContext.HttpContext.Session["LoginName"] == null || filterContext.HttpContext.Session["LoginName"].ToString()=="")
{
//HttpContext.Current.Response.Write("认证不通过");
//HttpContext.Current.Response.End();
filterContext.Result = new RedirectResult("/Login/Index");
}
}
}
}
通常 Authorize 过滤器也是在全局过滤器上面的,在 App_Start 目录下的 FilterConfig 类的 RegisterGlobalFilters 方法中添加:
using System.Web;
using System.Web.Mvc;
using MvcApp.Filter;
namespace MvcApp
{
public class FilterConfig
{
public static void RegisterGlobalFilters(GlobalFilterCollection filters)
{
filters.Add(new HandleErrorAttribute());
// 添加全局授权过滤器
filters.Add(new LoginAuthorizeAttribute());
}
}
}
Global.asax 下的代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Optimization;
using System.Web.Routing;
namespace MvcApp
{
public class MvcApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
AreaRegistration.RegisterAllAreas();
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
}
}
}
xoyozo:暂时没有按区域(Area)来统一配置的方法,建议加在 Controller 上。https://stackoverflow.com/questions/2319157/how-can-we-set-authorization-for-a-whole-area-in-asp-net-mvc
nuget 安装组件:MetadataExtractor
从文件流读取文件信息:
public IActionResult UploadFile([FromForm(Name = "[]")]IFormFile file)
{
var md = ImageMetadataReader.ReadMetadata(file.OpenReadStream());
var dic = new Dictionary<string, string>();
foreach (var m in md)
{
foreach (var t in m.Tags)
{
dic.Add(m.Name + " - " + t.Name, t.Description);
}
}
return Ok(dic);
}
结果演示:
从结果中可以看到计算的尺寸、拍摄设备、拍摄时间等信息。
MetadataExtractor 是一个简单而轻便的库,用于从图像和视频文件中读取元数据。
MetadataExtractor 从 JPEG、TIFF、WebP、PSD、PNG、BMP、GIF、ICO、PCX 和相机 RAW 文件读取 Exif、IPTC、XMP、ICC、Photoshop、WebP、PNG、BMP、GIF、ICO、PCX 元数据。
此外,还支持 MOV 和相关的 QuickTime 视频格式,例如 MP4、M4V、3G2、3GP。
相机制造商特定的支持包括爱克发,佳能,卡西欧,DJI,爱普生,富士胶片,柯达,京瓷,徕卡,美能达,尼康,奥林巴斯,松下,宾得,Recononyx,三洋,Sigma / Foveon 和索尼型号。
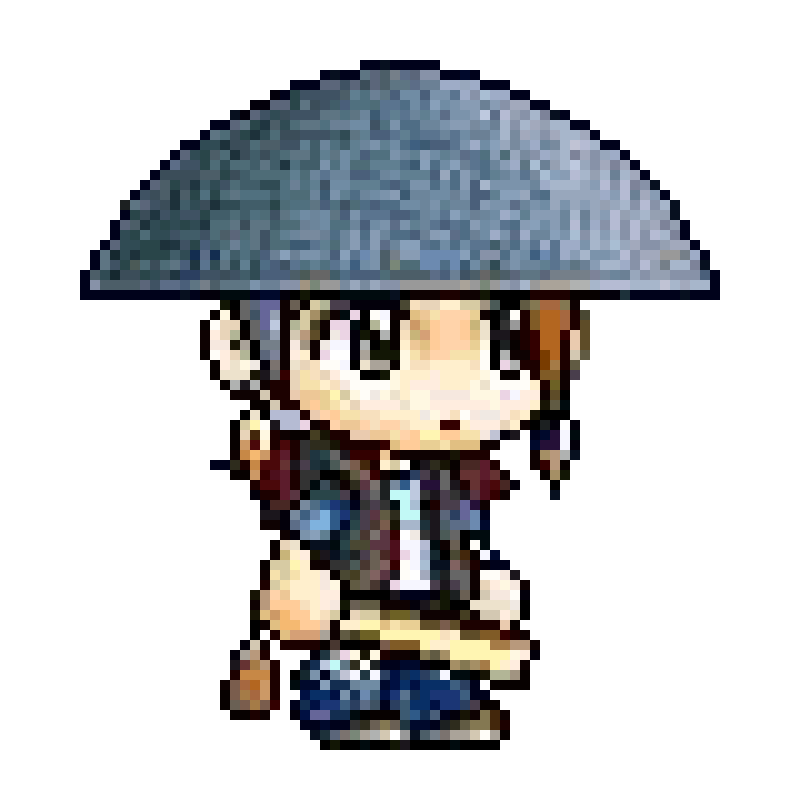
An unhandled exception occurred while processing the request.
InvalidOperationException: RenderBody has not been called for the page at '/Areas/Admin/Views/Shared/_Layout.cshtml'. To ignore call IgnoreBody().
Microsoft.AspNetCore.Mvc.Razor.RazorPage.EnsureRenderedBodyOrSections()
解决方法一:
在 return; 前执行 IgnoreBody();
解决方法二:
不要在 @RenderBody() 之前执行 return;
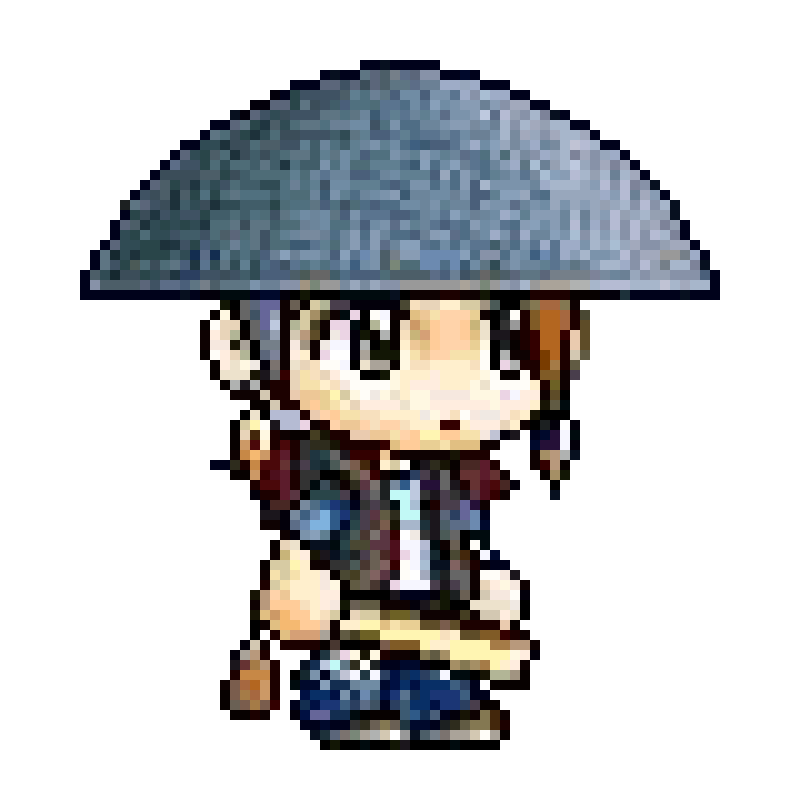
首先在项目根目录中创建目录 Areas。
在 Areas 中添加区域,以“Admin”、“Console”两个区域为例:
VS 提醒在 Startup.cs 的 Configure() 方法中添加 app.UseMvc,此与 app.UseEndpoints 冲突,我们改为在 app.UseEndpoints 中添加 MapControllerRoute(放在 default 之前):
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "MyArea",
pattern: "{area:exists}/{controller=Home}/{action=Index}/{id?}");
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
给区域的控制器添加属性,如:
[Area("Admin")]
public class HomeController : Controller
{
public IActionResult Index()
{
return View();
}
}
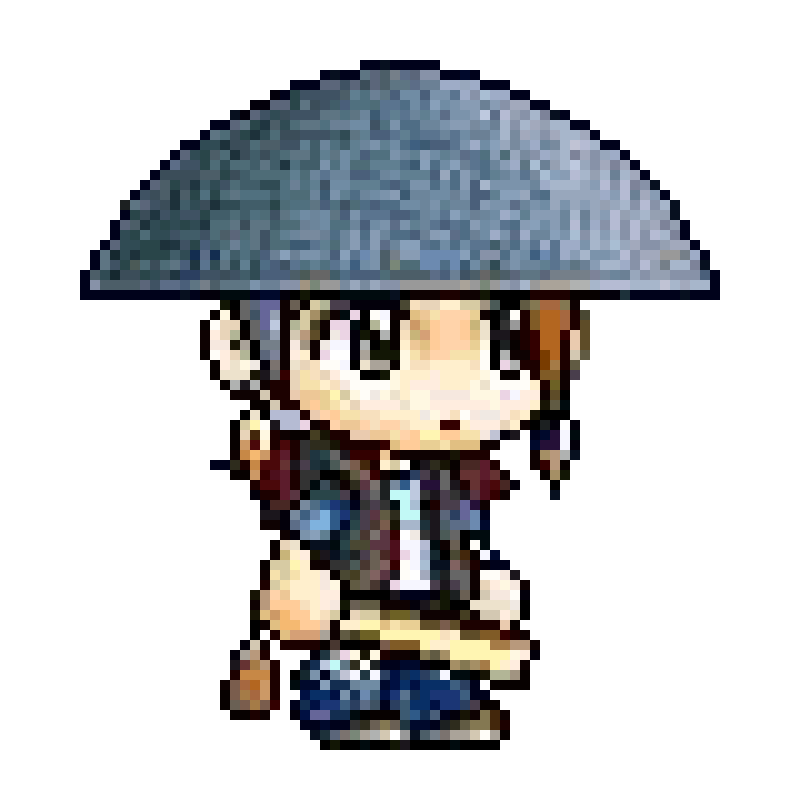